The BS mirror in LIGO is considered not large enough. Therefore, Hiro mentioned that there was a calculation long time ago about the clipping loss at BS. The calculation was done with OSCAR and FINESSE, however, different result was found. So we want to use these simulation tools to do these calculation again.
On my side, I am doing the FINESSE part. In FINESSE, we will need to use a phase map to apply a clear aperture. The way to create this phase map is
# create the aperture map for PR/SR/Input/End
diameter_m = 0.33
radius_m = diameter_m / 2
x_m = y_m = np.linspace(-radius_m, radius_m, 200)
smap_m = Map(
x_m,
y_m,
amplitude=circular_aperture(x_m, y_m, radius_m, x_offset=0.0, y_offset=0.0),
)
Then we use the following code to apply the created phase map to a mirror using the following code
localvirgo.model.PR.surface_map = smap_m
localvirgo.model.SR.surface_map = smap_m
localvirgo.model.NI.surface_map = smap_m
localvirgo.model.NE.surface_map = smap_m
localvirgo.model.WI.surface_map = smap_m
localvirgo.model.WE.surface_map = smap_m
For BS, the phase map should be applied in a different way since there is an incident angle. A elliptical aperture is created using the following code
def elliptical_aperture(x, y, R_ap, ang, x_offset=0, y_offset=0):
#x,y,R_ap is diameter in meter
m_ang = ang/180*np.pi
X = np.linspace(-x/2, x/2, 200)
Y = np.linspace(-y/2, y/2, 200)
xv, yv = np.meshgrid(X, Y)
R = np.sqrt((xv/np.sin(m_ang)) ** 2 + (yv) ** 2)
m = np.ones_like(xv)
m[R > R_ap/2] = 0
return X,Y,m
This code returns three arrays, the first one is X axis, the second one is Y axis, the third one is the map with 1 inside the aperture and 0 outside the aperture. To test this function, we run the following code
import matplotlib.pyplot as plt
from matplotlib import cbook
from matplotlib import cm
import colorcet as cc
from matplotlib.colors import LightSource
m = elliptical_aperture(0.55, 0.55, 0.55, 45)
fig, ax = plt.subplots(1, figsize=(8,8), dpi=100)
c = ax.pcolormesh(m[0], m[1], m[2], cmap=cc.cm.diverging_bwr_20_95_c54)
cbar = fig.colorbar(c, ax=ax)
cbar.set_label(r'Normalized spectrum density [A.U.]', fontsize=16)
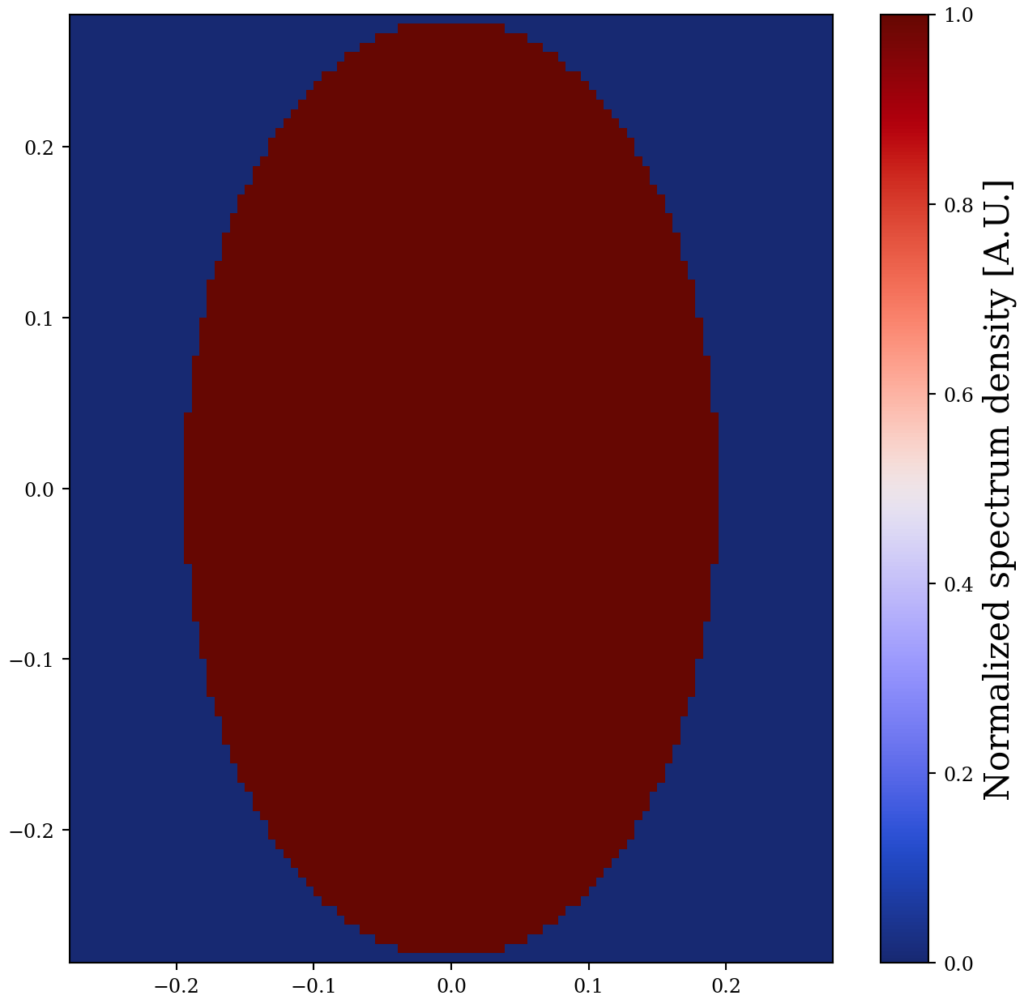
To apply this phase map to mirror, we did the following
# create the aperture map for BS
diameter_bs = 0.55
ang_bs = 45
radius_bs = diameter_bs / 2
x_bs = y_bs = np.linspace(-radius_bs, radius_bs, 200)
smap_bs = Map(
x_bs,
y_bs,
amplitude=elliptical_aperture(diameter_m, diameter_bs, diameter_bs, ang_bs, x_offset=0.0, y_offset=0.0)[2],
)
# apply to BS front surface
localvirgo.model.lpr_yu.surface_map = smap_bs
# apply to BS back surface
localvirgo.model.lsr_yu.surface_map = smap_bs